How to Use PowerShell's Get-Credential for Secure Authentication
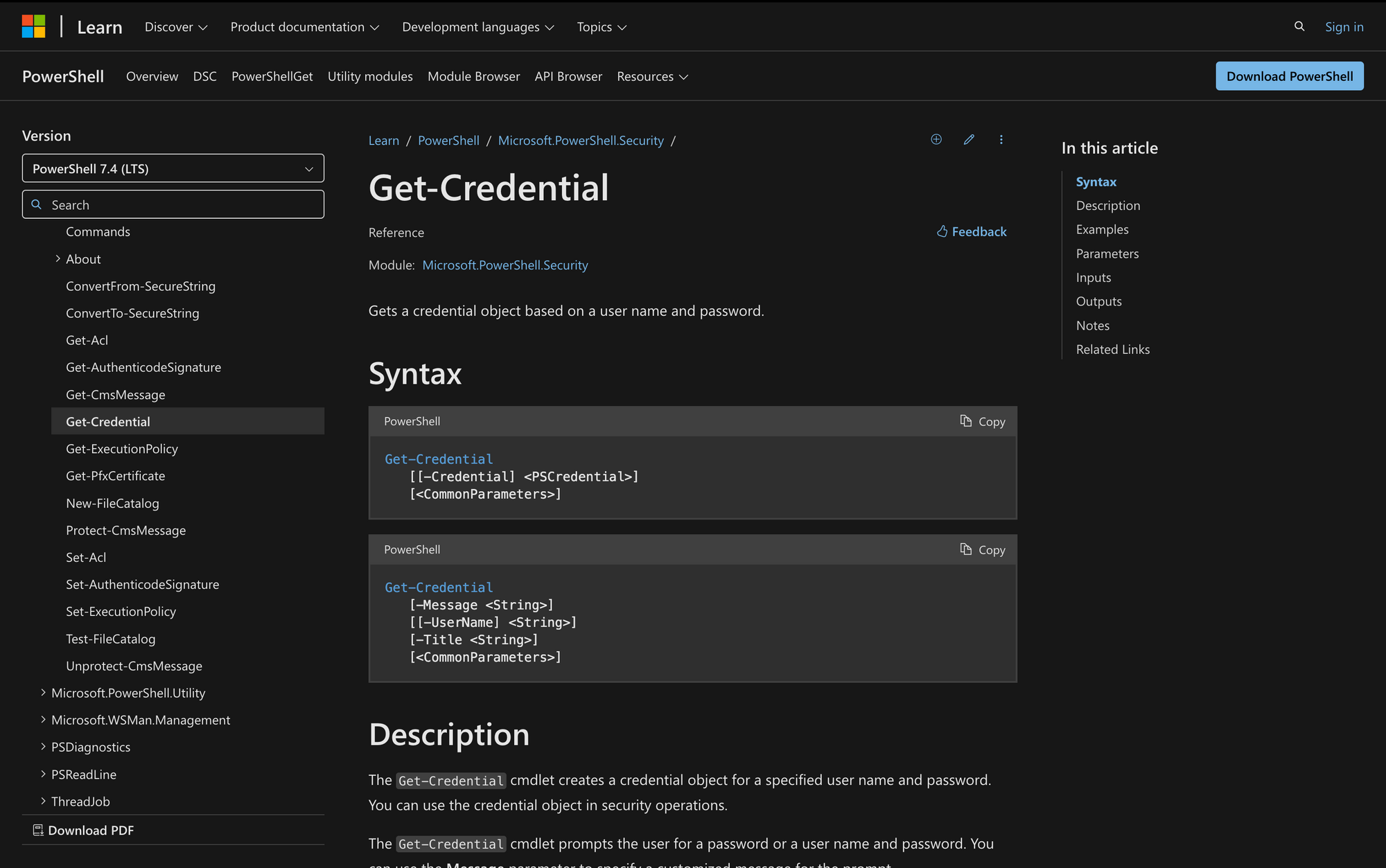
In the modern IT landscape, securely managing credentials is paramount for system administrators, IT professionals, and developers alike.
Through this guide, you'll learn the significance of the Get-Credential cmdlet, understand its syntax, and explore practical scenarios where it can save time and enhance security.
Whether connecting to remote systems, running scripts requiring authentication, or automating tasks with sensitive credentials, Get-Credential is a toolkit essential you need to know.
What is Get-Credential in PowerShell?
PowerShell's Get-Credential cmdlet is a function for securely request user authentication. It prompts users to enter credentials (username and password parameters) through a dialog box and encapsulates these credentials in a secure PSCredential object, which can be used within scripts and automation tasks.
Importantly, security credentials are never permanently stored, minimizing the risk of exposure in scripts. The cmdlet can also work with secure strings, ensuring that plain text passwords are not hard-coded into scripts.
The primary purpose of the Get-Credential cmdlet is to securely prompt users for credentials when required, minimizing the risk of exposing sensitive data directly within scripts.
Here is the basic syntax for using the Get-Credential cmdlet:
Get-Credential [-UserName] [-Message] [-Title]
In this syntax:
- UserName: Specifies the username for the credential parameter. If omitted, the prompt will ask for both the username and password.
- Message: Provides a custom message in the credential prompt, useful for giving users more context about why credentials are needed.
- Title: Specifies the title of the authentication prompt.
For example, to prompt the user with a custom message:
$credential = Get-Credential -Message "Credentials required for access to the \Server1\Fileshare." -UserName "Server01\PowerUser"
In this example, the command prompts the user to enter credentials with a message explaining the purpose of the request. These credentials can then be securely passed into other scripts or cmdlets.
Whether connecting to remote servers, accessing Exchange Online, or integrating with Azure services, Get-Credential helps ensure that authentication is secure, and credentials are never hard-coded into scripts, protecting sensitive information.
3 Common Use Cases for the Get-Credential Command
1. Connecting to Remote Servers
Use Get-Credential to securely gather and store credentials when connecting to remote servers. This practice ensures that sensitive information (such as usernames and passwords) is not exposed in scripts or logs.
For example, when using cmdlets like Enter-PSSession or Invoke-Command to access a remote server, you can pass the credential object returned by Get-Credential to securely authenticate the connection:
$credential = Get-Credential
Enter-PSSession -ComputerName Server01 -Credential $credential
2. Running Authentication-Required Scripts
Scripts that require elevated privileges or access to secured resources often need user authentication. Incorporating Get-Credential within such scripts prompts the user to provide credentials, which are securely passed during script execution.
This is commonly used for accessing services like Exchange Online or Azure AD:
$credential = Get-Credential
Connect-ExchangeOnline -Credential $credential
3. Automating Credential-Based Tasks
For tasks that are routinely automated but require authentication, Get-Credential helps manage the input of credentials securely.
While automation typically requires avoiding manual input, PowerShell also allows for the secure storage and retrieval of credentials using the Credential Manager or Export-Clixml and Import-Clixml for PSCredential objects.
This approach is key when automating scripts that require regular authentication:
$credential | Export-Clixml -Path "C:\secure-path\credentials.xml"
Prerequisites to Use the Get-Credential Cmdlet
To effectively use the Get-Credential cmdlet, ensure you meet the following prerequisites:
- Basic PowerShell Knowledge: Familiarity with PowerShell commands, scripting, and syntax is essential for effectively using Get-Credential. Understanding the PSCredential class and how to pass it into cmdlets like Invoke-Command, New-PSSession, or Connect-ExchangeOnline will ensure you can properly integrate Get-Credential into various tasks.
- Proper Permissions: You need appropriate permissions to perform tasks that require Get-Credential. This could range from administrative privileges for system-level tasks to permissions necessary for accessing specific remote systems, cloud services, or other secured environments.
How to Use Get-Credential Cmdlet in 5 Steps
1. Prompt for Credentials
Use the Get-Credential cmdlet to prompt the user for their credentials. In its basic form, this command opens a dialog box for the user to input a username and password:
$credential = Get-Credential
When executed, an external window will appear, allowing the user to securely input their credentials (e.g., username and password).
2. Store Credentials in a Variable
The credentials entered by the user are securely stored in a PSCredential object, which is assigned to the $credential variable. To verify the contents of the variable (such as the username), you can type:
$credential
This will display the PSCredential object. While the username is shown in plain text, the password is stored securely as a System.Security.SecureString and will not be visible, ensuring password visibility is limited.
3. Use Credentials with Other Cmdlets
Now you can use this new PSCredential object with cmdlets that support PSCredential object. You can pass the stored $credential to these cmdlets for remote connections or operations that require authentication. For example, to execute a command on a remote server:
Invoke-Command -ComputerName RemoteServer -Credential $credential -ScriptBlock { hostname }
This command uses the credentials stored in $credential to connect to RemoteServer and run the hostname command.
4. Specify a Custom Message
You can provide a custom message in the credential prompt to give users more context. This is especially useful in environments where users may need to supply specific credentials (e.g., domain admin credentials).
Use the -Message parameter to specify the message:
$credential = Get-Credential -Message "Please enter your domain account credentials"
This will display a prompt with the specified message, helping users understand which credentials to provide.
5. Save Credentials Securely (Optional)
For tasks that require automation, securely saving credentials is critical. Instead of storing credentials in plain text within scripts, you can use secure methods like the Windows Credential Manager or PowerShell's Export-Clixml and Import-Clixml cmdlets to save and retrieve credentials securely.
For example, you can export and import credentials using a secure XML file:
credential = Get-Credential
$credential | Export-Clixml -Path "C:\secure-path\mycredentials.xml"
# To retrieve the credentials later:
$credential = Import-Clixml -Path "C:\secure-path\mycredentials.xml"
Important: Ensure that only authorized users have access to the stored file, and avoid storing the credentials in unencrypted formats. Alternatively, you can use third-party secure vaults for better management of sensitive information.
Understanding and effectively using the Get-Credential cmdlet in PowerShell lets you handle authentication securely and efficiently.
By integrating this powerful tool into your workflow, you can ensure that credentials are managed responsibly, reducing security risks while streamlining system administration tasks. Adopt these best practices and improve your scripts' reliability and security.